What Is Web Crypto API? Exploring the Basics of Web Cryptography APIs
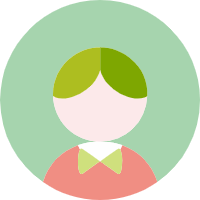
The Web Crypto API (Web Cryptography API) is a JavaScript API that provides access to secure computing capabilities, such as cryptography, encryption, and hash functions, within the browser. It aims to make it easier for developers to use cryptographic methods in their web applications, while also providing a safe and secure environment for users. In this article, we will explore the basics of the Web Crypto API, its purpose, and how it can be used in web applications.
Purpose of the Web Crypto API
The Web Crypto API was introduced in Chrome 28 and is now supported by most popular browsers, including Chrome, Firefox, and Edge. It provides a way for developers to perform secure computing tasks, such as encryption, decryption, hash calculations, and more, without having to worry about the security implications of using these methods.
By providing a standardized API for cryptography, the Web Crypto API aims to make it easier for developers to implement secure communication between browsers and web applications, as well as to protect sensitive data stored within the browser.
Basics of the Web Crypto API
The Web Crypto API is divided into several components, each with its own purpose and functionality. Here are some of the basic concepts and methods you should be familiar with when working with the Web Crypto API:
1. CryptoKey: A CryptoKey represents a secret value, such as a cryptographic key, that is used in cryptographic operations. There are two types of CryptoKey: CryptoKeyPair, which represents a pair of publicly and privately encrypted keys, and CryptoKeyFormat, which represents the format of the key, such as ecdsa, rsa, etc.
2. CryptoBuffer: A CryptoBuffer is a data structure that can be used to store binary data, such as encrypted data or hashes, within the Web Crypto API. It is designed to be used with CryptoOperations to perform cryptographic tasks.
3. CryptoOperation: A CryptoOperation represents a specific cryptographic operation, such as encryption, decryption, hash calculation, etc. It contains a list of input and output CryptoBuffers, as well as any optional parameters needed for the operation.
4. Subkey: A Subkey is a derivative of a CryptoKey that represents a portion of the original key. It can be used for partial encryption or decryption tasks.
5. Sign and Verify: These methods are used for digital signature and verification tasks. They involve the use of a PrivateKey and a CryptoBuffer containing the data to be signed or verified.
How to Use the Web Crypto API in Web Applications
The Web Crypto API can be used in various web applications, such as server-side applications, mobile applications, and more. Here's an example of how to use the Web Crypto API to encrypt and decrypt data using a CryptoKeyPair in a JavaScript environment:
```javascript
// Create a new CryptoKeyPair
const keyPair = webcrypto.generateKeyPair('ecdsa', { name: 'P-256' });
// Encrypt some data using the public key
const publicKey = keyPair.publicKey;
const dataToEncrypt = 'Some encrypted data';
const encryptedData = publicKey.encrypt(dataToEncrypt);
// Decrypt the encrypted data using the private key
const privateKey = keyPair.privateKey;
const decryptedData = privateKey.decrypt(encryptedData);
console.log('Encrypted data:', encryptedData);
console.log('Decrypted data:', decryptedData);
```
In this example, we created a new CryptoKeyPair using the webcrypto.generateKeyPair() method and then used the public and private keys to encrypt and decrypt some data.
The Web Crypto API provides a powerful and secure way for developers to perform cryptographic tasks within the browser. By understanding its basics and using it in web applications, developers can create more secure and private experiences for their users. As the Web Crypto API continues to evolve and improve, it is essential for developers to stay up-to-date with its latest features and best practices.